Digram of the data flow. |
With my new pipeline sketched out, it was pretty straightforward to code up a handler to listen for incoming tweets, transform them, and re-tweet any statuses the model thinks are really from Trump. I deployed everything to an EC2 instance, loaded a serialized model (so the feature processing Pipeline came along for free) and just waited for Donald Trump to get in a bad mood.
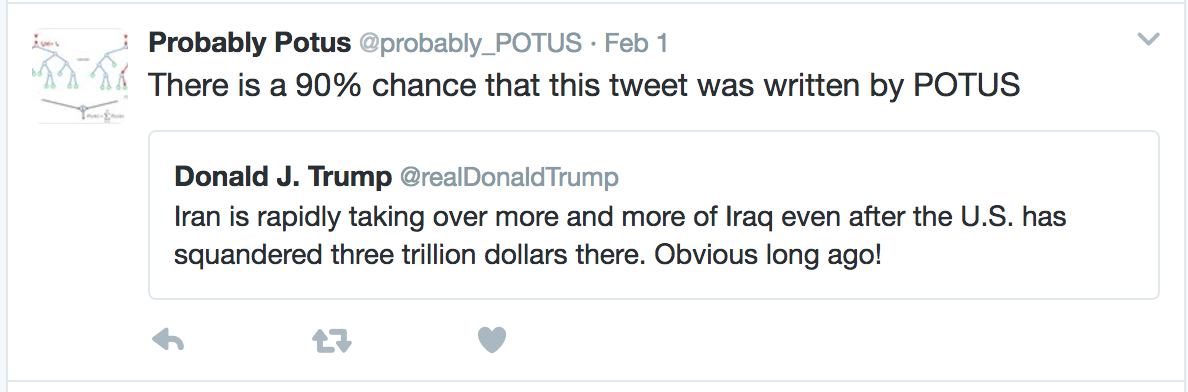 |
Success! |
The bot has been running for a few days now and it seems to be working pretty well. There are a few issues I'd like to tackle to improve it, so I'll probably keep tweaking it for some time. In the meantime, follow along with @Probably_POTUS. Be careful, though...these tweets are the craziest of the crazy.
The entire code for this project can be found on GitHub. Feedback or PRs are more than welcome!
Leave a Comment